1.jsp文件
<head>
<link href="${path}/assets/js/kindeditor/themes/default/default.css"/>
<script src="${path}/assets/js/kindeditor/kindeditor-all.js"></script>
<script src="${path}/assets/js/kindeditor/lang/zh-CN.js"></script>
</head>
<label id="article_cnt_limit">内容</label>
<div class="clearfix">
<textarea type="textarea" id="template_text" maxlength="500" class="form-control"
style="height: 100px; resize: none"></textarea>
</div>
2.js文件
var editor;
//页面加载初始化富文本
$(document).ready(function() {
editor = getKindEditor("template_text","article_cnt_limit")
});
// 获取富文本的内容,可以显示在页面上
params['content'] = editor.html()
//设置只读模式的时候,图片和表格不可操作
KindEditor.ready(function(K) {
editor.readonly(true);
var iframe = K.query("iframe");
//查看时禁止鼠标右击
var img = $(iframe).contents().find("img");
img.bind("contextmenu",function(e){
return false;
});
//查看时禁止鼠标右击
var table = $(iframe).contents().find("table");
table.bind("contextmenu",function(e){
return false;
});
});
function getKindEditor(textId,labelId,callbackFunc){
//富文本编辑器
KindEditor.ready(function(K) {
editor = K.create('#'+textId, {
filterMode : false,
resizeType : 1,height:"630px",
//上传的配置
uploadJson : path + "/appletManage/kindEditor/fileUpload",
fileManagerJson : path + "/appletManage/kindEditor/fileManager",
allowFileManager : true,
formatUploadUrl: false,
//字数限制
afterChange : function(){
//限制字数
var limitNum = 10000; //设定限制字数
var pattern = '还可以输入' + limitNum + '字';
$('#'+labelId).html(pattern); //输入显示
if (this.count('text') > limitNum) {
pattern = ('字数超过限制,请适当删除部分内容');
//超过字数限制自动截取
var strValue = editor.text();
strValue = strValue.substring(0, limitNum);
editor.text(strValue);
} else {
//计算剩余字数
var result = limitNum - this.count('text');
pattern = '还可以输入' + result + '字';
}
$('#'+labelId).html(pattern); //输入显示
}
});
if(callbackFunc){
callbackFunc();
}
});
}
3.Java类
package com.ctbr.applet.controller.manage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Random;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItemFactory;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import com.ctbr.applet.util.AppletUtils;
import com.ctbr.baseFrame.core.BaseController;
import com.ctbr.baseFrame.util.Const;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.io.ByteStreams;
/**
* 富文本图片上传
*/
@Controller
@RequestMapping(value = "/appletManage/kindEditor")
public class KindEditorController extends BaseController {
private static final ObjectMapper objectMapper = new ObjectMapper();
@RequestMapping(value = "/fileUpload", method = RequestMethod.POST)
@ResponseBody
public Map<String, Object> fileUpload(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException, FileUploadException {
String savePath = Const.LOCAL_CONTENT_PATH + "kindEditor/";
// 文件保存目录URL
String saveUrl = AppletUtils.getPathContent() + "/kindEditor/";
// 定义允许上传的文件扩展名
HashMap<String, String> extMap = new HashMap<String, String>();
extMap.put("image", "gif,jpg,jpeg,png,bmp");
extMap.put("flash", "swf,flv");
extMap.put("media", "swf,flv,mp3,mp4,wav,wma,wmv,mid,avi,mpg,asf,rm,rmvb");
extMap.put("file", "doc,docx,xls,xlsx,ppt,htm,html,txt,zip,rar,gz,bz2");
response.setContentType("text/html; charset=UTF-8");
if (!ServletFileUpload.isMultipartContent(request)) {
return getError("请选择文件。");
}
// 检查目录
File uploadDir = new File(savePath);
if (!uploadDir.isDirectory()) {
// return getError("上传目录不存在。");
uploadDir.mkdirs();
}
// 检查目录写权限
if (!uploadDir.canWrite()) {
return getError("上传目录没有写权限。");
}
String dirName = request.getParameter("dir");
if (dirName == null) {
dirName = "image";
}
if (!extMap.containsKey(dirName)) {
return getError("目录名不正确。");
}
// 创建文件夹
savePath += dirName + "/";
saveUrl += dirName + "/";
File saveDirFile = new File(savePath);
if (!saveDirFile.exists()) {
saveDirFile.mkdirs();
}
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
String ymd = sdf.format(new Date());
savePath += ymd + "/";
saveUrl += ymd + "/";
File dirFile = new File(savePath);
if (!dirFile.exists()) {
dirFile.mkdirs();
}
FileItemFactory factory = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(factory);
upload.setHeaderEncoding("UTF-8");
// 最大文件大小
long imageMaxSize = 1048576;
long flashMaxSize = 5*1048576;
long mediaMaxSize = 20*1048576;
long fileMaxSize = 5*1048576;
MultipartHttpServletRequest multipartRequest = (MultipartHttpServletRequest) request;
Iterator<String> item = multipartRequest.getFileNames();
while (item.hasNext()) {
String fileName = item.next();
MultipartFile file = multipartRequest.getFile(fileName);
// 检查文件大小
if("image".equals(dirName)) {
if (file.getSize() > imageMaxSize) {
return getError("上传文件大小超过限制,限制图片为1M。");
}
} else if("flash".equals(dirName)) {
if (file.getSize() > flashMaxSize) {
return getError("上传文件大小超过限制,限制Flash为5M。");
}
} else if("media".equals(dirName)) {
if (file.getSize() > mediaMaxSize) {
return getError("上传文件大小超过限制,限制视频为20M。");
}
} else if("file".equals(dirName)) {
if (file.getSize() > fileMaxSize) {
return getError("上传文件大小超过限制,限制文件为5M。");
}
} else {
if (file.getSize() > imageMaxSize) {
return getError("上传文件大小超过限制,限制为1M。");
}
}
// 检查扩展名
String fileExt = file.getOriginalFilename().substring(file.getOriginalFilename().lastIndexOf(".") + 1)
.toLowerCase();
if (!Arrays.asList(extMap.get(dirName).split(",")).contains(fileExt)) {
return getError("上传文件扩展名是不允许的扩展名。\n只允许" + extMap.get(dirName) + "格式。");
}
SimpleDateFormat df = new SimpleDateFormat("yyyyMMddHHmmss");
String newFileName = df.format(new Date()) + "_" + new Random().nextInt(1000) + "." + fileExt;
try {
File uploadedFile = new File(savePath, newFileName);
ByteStreams.copy(file.getInputStream(), new FileOutputStream(uploadedFile));
} catch (Exception e) {
return getError("上传文件失败。");
}
Map<String, Object> msg = new HashMap<String, Object>();
msg.put("error", 0);
msg.put("url", saveUrl + newFileName);
return msg;
}
return null;
}
private Map<String, Object> getError(String message) {
Map<String, Object> msg = new HashMap<String, Object>();
msg.put("error", 1);
msg.put("message", message);
return msg;
}
@SuppressWarnings("unchecked")
@RequestMapping(value = "/fileManager", method = RequestMethod.GET)
public void fileManager(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
ServletOutputStream out = response.getOutputStream();
// 根目录路径,可以指定绝对路径,比如 /var/www/attached/
String rootPath = Const.LOCAL_CONTENT_PATH + "kindEditor/";
// 根目录URL,可以指定绝对路径,比如 http://www.yoursite.com/attached/
String rootUrl = AppletUtils.getPathContent() + "/kindEditor/";
// 图片扩展名
String[] fileTypes = new String[] { "gif", "jpg", "jpeg", "png", "bmp" };
String dirName = request.getParameter("dir");
if (dirName != null) {
if (!Arrays.<String>asList(new String[] { "image", "flash", "media", "file" }).contains(dirName)) {
out.println("Invalid Directory name.");
return;
}
rootPath += dirName + "/";
rootUrl += dirName + "/";
File saveDirFile = new File(rootPath);
if (!saveDirFile.exists()) {
saveDirFile.mkdirs();
}
}
// 根据path参数,设置各路径和URL
String path = request.getParameter("path") != null ? request.getParameter("path") : "";
String currentPath = rootPath + path;
String currentUrl = rootUrl + path;
String currentDirPath = path;
String moveupDirPath = "";
if (!"".equals(path)) {
String str = currentDirPath.substring(0, currentDirPath.length() - 1);
moveupDirPath = str.lastIndexOf("/") >= 0 ? str.substring(0, str.lastIndexOf("/") + 1) : "";
}
// 排序形式,name or size or type
String order = request.getParameter("order") != null ? request.getParameter("order").toLowerCase() : "name";
// 不允许使用..移动到上一级目录
if (path.indexOf("..") >= 0) {
out.println("Access is not allowed.");
return;
}
// 最后一个字符不是/
if (!"".equals(path) && !path.endsWith("/")) {
out.println("参数无效。");
return;
}
// 目录不存在或不是目录
File currentPathFile = new File(currentPath);
if (!currentPathFile.isDirectory()) {
out.println("目录不存在。");
return;
}
// 遍历目录取的文件信息
List<Hashtable<String, Object>> fileList = new ArrayList<Hashtable<String, Object>>();
if (currentPathFile.listFiles() != null) {
for (File file : currentPathFile.listFiles()) {
Hashtable<String, Object> hash = new Hashtable<String, Object>();
String fileName = file.getName();
if (file.isDirectory()) {
hash.put("is_dir", true);
hash.put("has_file", (file.listFiles() != null));
hash.put("filesize", 0L);
hash.put("is_photo", false);
hash.put("filetype", "");
} else if (file.isFile()) {
String fileExt = fileName.substring(fileName.lastIndexOf(".") + 1).toLowerCase();
hash.put("is_dir", false);
hash.put("has_file", false);
hash.put("filesize", file.length());
hash.put("is_photo", Arrays.<String>asList(fileTypes).contains(fileExt));
hash.put("filetype", fileExt);
}
hash.put("filename", fileName);
hash.put("datetime", new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(file.lastModified()));
fileList.add(hash);
}
}
if ("size".equals(order)) {
Collections.sort(fileList, new SizeComparator());
} else if ("type".equals(order)) {
Collections.sort(fileList, new TypeComparator());
} else {
Collections.sort(fileList, new NameComparator());
}
Map<String, Object> msg = new HashMap<String, Object>();
msg.put("moveup_dir_path", moveupDirPath);
msg.put("current_dir_path", currentDirPath);
msg.put("current_url", currentUrl);
msg.put("total_count", fileList.size());
msg.put("file_list", fileList);
response.setContentType("application/json; charset=UTF-8");
String msgStr = objectMapper.writeValueAsString(msg);
out.println(msgStr);
}
@SuppressWarnings("rawtypes")
class NameComparator implements Comparator {
public int compare(Object a, Object b) {
Hashtable hashA = (Hashtable) a;
Hashtable hashB = (Hashtable) b;
if (((Boolean) hashA.get("is_dir")) && !((Boolean) hashB.get("is_dir"))) {
return -1;
} else if (!((Boolean) hashA.get("is_dir")) && ((Boolean) hashB.get("is_dir"))) {
return 1;
} else {
return ((String) hashA.get("filename")).compareTo((String) hashB.get("filename"));
}
}
}
@SuppressWarnings("rawtypes")
class SizeComparator implements Comparator {
public int compare(Object a, Object b) {
Hashtable hashA = (Hashtable) a;
Hashtable hashB = (Hashtable) b;
if (((Boolean) hashA.get("is_dir")) && !((Boolean) hashB.get("is_dir"))) {
return -1;
} else if (!((Boolean) hashA.get("is_dir")) && ((Boolean) hashB.get("is_dir"))) {
return 1;
} else {
if (((Long) hashA.get("filesize")) > ((Long) hashB.get("filesize"))) {
return 1;
} else if (((Long) hashA.get("filesize")) < ((Long) hashB.get("filesize"))) {
return -1;
} else {
return 0;
}
}
}
}
@SuppressWarnings("rawtypes")
class TypeComparator implements Comparator {
public int compare(Object a, Object b) {
Hashtable hashA = (Hashtable) a;
Hashtable hashB = (Hashtable) b;
if (((Boolean) hashA.get("is_dir")) && !((Boolean) hashB.get("is_dir"))) {
return -1;
} else if (!((Boolean) hashA.get("is_dir")) && ((Boolean) hashB.get("is_dir"))) {
return 1;
} else {
return ((String) hashA.get("filetype")).compareTo((String) hashB.get("filetype"));
}
}
}
}
4.效果图
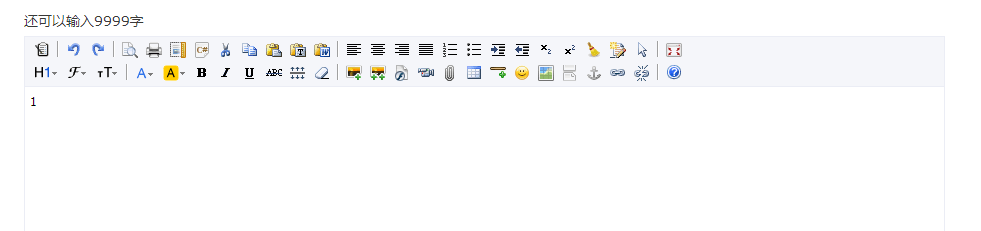
5.官方文档
链接地址
评论